Welcome
Press the Right Arrow
SeleniumBase Presenter
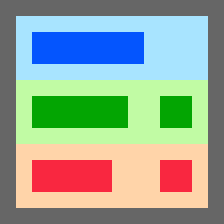
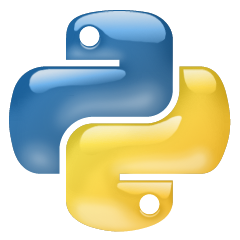
Create presentations with Python
Make slides using HTML:
Row ABC | Row XYZ |
Value ONE | Value TWO |
Value THREE | Value FOUR |
(HTML table example)
Keyboard Shortcuts:
Key | Action |
=> | Next Slide (N also works) |
<= | Previous Slide (P also works) |
F | Full Screen Mode |
O | Overview Mode Toggle |
esc | Exit Full Screen / Overview Mode |
. | Pause/Resume Toggle |
space | Next Slide (alternative) |
Add code to slides:
from seleniumbase import BaseCase
class MyTestClass(BaseCase):
def test_basics(self):
self.open("https://xkcd.com/353/")
self.assert_title("xkcd: Python")
self.assert_element('img[alt="Python"]')
self.click('a[rel="license"]')
self.assert_text("free to copy and reuse")
self.go_back()
self.click_link("About")
self.assert_exact_text("xkcd.com", "h2")
Highlight code in slides:
from seleniumbase import BaseCase
class MyTestClass(BaseCase):
def test_basics(self):
self.open("https://xkcd.com/353/")
self.assert_title("xkcd: Python")
self.assert_element('img[alt="Python"]')
self.click('a[rel="license"]')
self.assert_text("free to copy and reuse")
self.go_back()
self.click_link("About")
self.assert_exact_text("xkcd.com", "h2")
Getting started is easy:
from seleniumbase import BaseCase
class MyPresenterClass(BaseCase):
def test_presenter(self):
self.create_presentation(theme="serif")
self.add_slide("Welcome to Presenter!")
self.add_slide(
"Add code to slides:",
code=(
"from seleniumbase import BaseCase\n\n"
"class MyPresenterClass(BaseCase):\n\n"
" def test_presenter(self):\n"
" self.create_presentation()\n"))
self.begin_presentation(
filename="demo.html", show_notes=True)
Include notes with slides:
self.add_slide("[Your HTML goes here]",
code="[Your software code goes here]",
content2="[Additional HTML goes here]",
notes="[Attached speaker notes go here]"
"[Note A! -- Note B! -- Note C! ]")
(Notes can include HTML tags)
Multiple themes available:
self.create_presentation(theme="serif")
self.create_presentation(theme="sky")
self.create_presentation(theme="simple")
self.create_presentation(theme="white")
self.create_presentation(theme="moon")
self.create_presentation(theme="black")
self.create_presentation(theme="night")
self.create_presentation(theme="beige")
self.create_presentation(theme="league")